Handling Network Retries
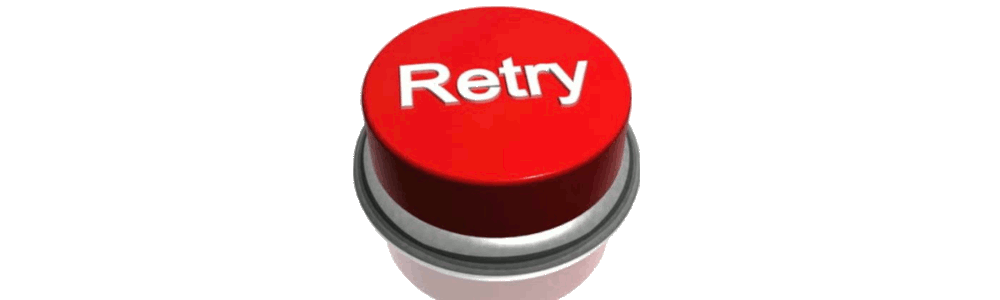
In a distributed environment, network retries are an essential mechanism to handle temporary failures in communication between different components. When using Docker containers, network retries can help to ensure the reliability and availability of containerized applications.
Network retries involve attempting a failed network operation multiple times until a successful response is received. This can be achieved through various approaches, including:
Retry within the application code: This involves adding retry logic within the application code to handle network failures. The application code can implement retries using libraries such as retrying or resilience.
External retry services: An external service, such as Apache ZooKeeper or Hashicorp Consul, can be used to coordinate retries across multiple containers. These services can detect when a container is down and redirect traffic to healthy containers.
Load balancers: Load balancers can also implement network retries by redirecting traffic to healthy containers when a container is down. Load balancers can also monitor the health of containers and perform automatic failover to healthy containers.
In Docker containers, network retries can be particularly useful when deploying applications that consist of multiple container instances. For example, if a containerized application has three instances of a web server, and one of them fails, network retries can help to ensure that requests are redirected to the other two instances until the failed instance is restored. This can improve the overall reliability and availability of the application.
In summary, network retries are an essential mechanism to ensure the reliability of containerized applications. Docker containers can implement network retries through various approaches, including retrying within the application code, external retry services, and load balancers.
Bash+curl implementation
|
|
Only curl in one line
Check flags with https://explainshell.com
|
|