List Bash Variables By Name
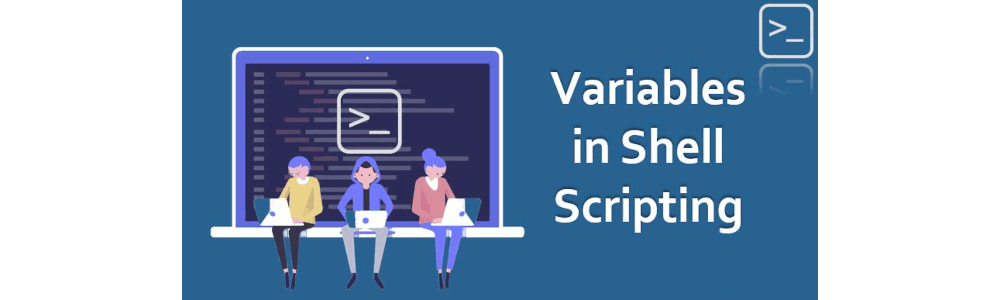
Bash script variables and environment variables are both used in Bash scripting, but they have different scopes and lifetimes.
A Bash script variable is a variable that is defined and used within a Bash script. It has a local scope and is accessible only within the script. Bash script variables are usually created using the = operator, and their values can be changed within the script. They are not visible outside of the script, and any changes made to them within the script do not affect the environment.
On the other hand, an environment variable is a variable that is set in the environment where a process runs. It has a global scope and can be accessed by any process that is running in that environment, including Bash scripts. Environment variables are usually created using the export command, and their values can be changed or read by any process that is running in that environment. Changes made to an environment variable affect all processes running in that environment.
One important difference between Bash script variables and environment variables is their lifetimes. Bash script variables exist only as long as the script is running, and they are destroyed when the script exits. Environment variables, on the other hand, persist even after the process that created them has terminated, and they can be used by other processes that are started later.
In summary, Bash script variables have a local scope and are used within a Bash script, while environment variables have a global scope and are accessible by any process running in that environment. Bash script variables have a limited lifetime, while environment variables persist after the process that created them has terminated.
Show local variables starts with ABC_
Script:
|
|
Output:
|
|
Show environment variables starts with ABC_
Script:
|
|
Output:
|
|